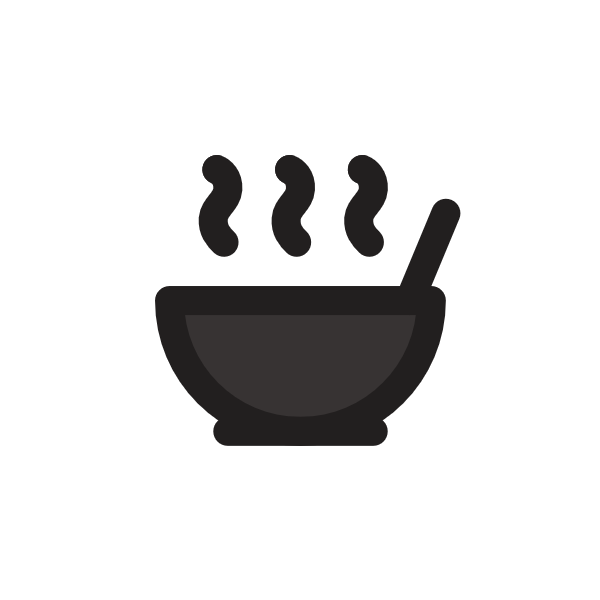
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
SQL injection
Read the original article here.
Okay, here is the detailed educational resource on SQL Injection, reframed within the context of "The Forbidden Code: Underground Programming Techniques They Won’t Teach You in School."
SQL Injection: Exploiting the Database Backdoor
Welcome to "The Forbidden Code," where we explore programming techniques that lie outside the standard curriculum, often venturing into the realm of system vulnerabilities and exploitation. Today, we delve into one of the oldest, most prevalent, and potentially devastating vulnerabilities in data-driven applications: SQL Injection. While schools teach you how to build applications that interact with databases, they often skim over or simply warn against the dangers lurking when user input isn't handled with extreme prejudice. Understanding SQL injection is crucial, not just for learning how attackers breach systems, but more importantly, for mastering how to build truly secure software.
1. What is SQL Injection?
At its core, SQL Injection (SQLI) is a method attackers use to interfere with the queries an application makes to its database. By inserting malicious code into input fields, an attacker can trick the database into executing commands they never intended, often with severe consequences.
Definition: SQL Injection (SQLI) is a code injection technique where an attacker provides malicious SQL statements as input to an application, exploiting vulnerabilities in how the application constructs and executes database queries.
This vulnerability typically arises when applications build SQL query strings by concatenating user-supplied data directly into the query without proper sanitization or validation. This allows the attacker's input to escape its intended role as data and be interpreted as executable code by the database.
While most commonly associated with web applications due to their public accessibility, SQL Injection can affect any application that interacts with a database using inadequately handled user input. This includes desktop applications, mobile apps, and internal tools. Even some document-oriented NoSQL databases can be susceptible to injection-like attacks if their query mechanisms process untrusted input unsafely.
Potential Consequences:
Exploiting SQL Injection can grant attackers a wide range of destructive capabilities:
- Identity Spoofing: Logging in as other users or administrators.
- Data Tampering: Modifying existing records (e.g., changing balances, altering credentials).
- Repudiation Issues: Voiding transactions, deleting logs.
- Complete Data Disclosure: Dumping the entire contents of the database, including sensitive information like user credentials, personal data, and business secrets.
- Data Destruction or Unavailability: Dropping tables, deleting data, or corrupting the database structure, leading to denial of service.
- Database Server Takeover: In some cases, gaining administrative access to the database server itself, potentially leading to broader system compromise.
The Open Web Application Security Project (OWASP) consistently ranks Injection vulnerabilities, including SQLI, among the most critical risks facing web applications. This persistent threat underscores the vital importance of understanding its mechanics and defenses.
2. A Brief History of the "Forbidden Art"
Discussions around the potential for injecting code into data inputs date back to the early days of databases and applications interacting via dynamic queries. SQL injection specifically gained prominence in the late 1990s, notably being detailed in a 1998 issue of Phrack Magazine, a long-standing online publication focused on hacking and security.
Its significance grew as web applications became ubiquitous. OWASP recognized SQL injection as a major threat early on, placing it in their initial Top 10 lists in the late 2000s. By 2013, it was listed as the single most critical web application vulnerability.
Over time, while specific techniques evolved and the category broadened to include other types of injection (OS command, LDAP, etc.), SQL injection remained a core component of this threat landscape. Even by 2021, injection vulnerabilities were still found in a vast majority of tested applications, highlighting a persistent failure in fundamental secure coding practices despite decades of awareness. The definition of 'injection' expanded to include newer technologies like ORMs, Expression Languages (EL), and Object Graph Navigation Library (OGNL), showing that the core principle – treating untrusted data as code – remains a universal vulnerability pattern.
3. The Root Cause: Data Escaping Its Bounds
To truly understand SQL injection, you must grasp the fundamental problem: the confusion between data and code. Database queries are constructed using specific commands (like SELECT
, INSERT
, UPDATE
, DELETE
) and operators (WHERE
, AND
, OR
, =
). These commands operate on data stored within the database.
The vulnerability occurs when an application builds an SQL query by simply stitching together parts of the query string with user-provided input using string concatenation. When the user input isn't properly handled, an attacker can craft input that includes not just data, but characters that have special meaning in SQL, effectively injecting new commands or altering the logic of the original command.
Consider a simple scenario: an application queries a users
table to find a user by their provided username. The developer might construct the SQL query like this (using pseudocode):
sql_query = "SELECT * FROM users WHERE username = '" + user_input + "';"
If a legitimate user enters "alice"
, the query becomes:
SELECT * FROM users WHERE username = 'alice';
This query works as intended, retrieving Alice's information.
Now, let's look at how an attacker exploits this. They understand that the application is inserting their input directly into the string delimited by single quotes ('
). They can use a single quote in their input to close the string the application started, and then add their own SQL commands.
Imagine the attacker enters the following as the username:
' OR '1'='1'; --
Let's break down this malicious input:
'
: This single quote closes the string started by the application before theuser_input
.OR '1'='1'
: This injects a new condition into theWHERE
clause. The condition1'='1
is always true. TheOR
operator means the overallWHERE
clause will be true if either the original condition is met or1'='1
is true. Since1'='1
is always true, the entireWHERE
clause becomes true, regardless of the username entered before theOR
.;
: This character is used to terminate an SQL statement. By adding it, the attacker can potentially execute multiple SQL commands within a single request if the database driver/API allows it (though many APIs, like PHP's legacymysql_query()
, prevent this multi-statement execution for basic queries).--
: This is the standard syntax for a single-line comment in many SQL dialects (including MySQL and SQL Server). Everything after--
on the same line is ignored by the database. The attacker uses this to comment out the rest of the original query string that the application would have added (in our example, the final'
and;
).
Combining the application's query fragments with the attacker's input:
Original fragments: "SELECT * FROM users WHERE username = '"
+ user_input
+ "';"
Attacker's input: ' OR '1'='1'; --
Resulting SQL query executed by the database:
SELECT * FROM users WHERE username = '' OR '1'='1'; --';
Because the --
comments out the trailing '
, the effective query is:
SELECT * FROM users WHERE username = '' OR '1'='1';
Since '1'='1'
is always true, the WHERE
clause evaluates to true for every row in the users
table. Instead of retrieving a single user by a specific username, the application's query now selects all users. If this query was used for authentication (e.g., checking if a user exists and then verifying a password - although the password check is often skipped if any user is returned), the attacker could potentially gain access without needing a valid username or password. This is a classic example of bypassing authentication.
This example clearly illustrates the core vulnerability: the attacker's input, intended as data (' OR '1'='1'; --
), is misinterpreted by the application's query builder and the database as code, altering the intended logic.
4. Advanced Exploitation Techniques
While the basic ' OR '1'='1
trick is fundamental, attackers have developed more sophisticated techniques to exploit SQL injection vulnerabilities, especially when direct output is not available.
4.1. Direct Output or Action (Classic Injection)
As seen in the basic example, sometimes the attacker can directly manipulate the query to:
- Retrieve Data: Use
UNION
clauses to combine the results of the original query with results from other tables (like theusers
table or system tables containing database schema information). For example, if the original query returns one row with two columns, an attacker might try:
If the number of columns matches and the data types are compatible, the database will return the usernames and passwords from the' UNION SELECT username, password FROM users; --
users
table mixed with the original query's results. This is a powerful technique for dumping data. - Execute Arbitrary Commands: If the API allows multiple statements (e.g., using
;
to separate commands), attackers can inject completely new, potentially destructive commands.
This input would close the original query, execute a'; DROP TABLE users; --
DROP TABLE
command to delete theusers
table, and then comment out the rest. If the database user account the application uses has permission to drop tables, the attacker just wiped your user data. Other commands could be injected, like inserting new administrative users or modifying existing data.Note: Many modern database APIs (like PHP's
mysqli
or prepared statements in various languages) are designed to prevent executing multiple statements passed as a single string, mitigating the risk of arbitrary command injection via;
. However, they do not prevent the modification of the original query's logic (like theOR '1'='1'
example) if parameterized statements aren't used.
4.2. Blind SQL Injection
Sometimes, exploiting SQL injection doesn't yield direct database output on the application's webpage or interface. For instance, a vulnerable parameter might be used in a query that only determines whether to display a specific page element or whether an action succeeds, without showing the retrieved data or error messages. This is where Blind SQL Injection comes into play.
Definition: Blind SQL Injection is an SQL injection technique used when the application does not display the results of the database query, but the attacker can infer information based on the application's behavior or response time, which is influenced by the injected SQL statement's outcome.
In blind SQLI, attackers craft queries that ask the database true/false questions. The application's different responses (e.g., page loads normally vs. page shows an error or is blank; page loads quickly vs. page takes a long time) act as the "signal" indicating whether the injected logical statement was true or false.
Techniques within Blind SQLI:
Boolean-Based Blind: This is the most common form. The attacker injects conditions that evaluate to true or false and observes the application's response.
- Example: Imagine a URL like
https://example.com/item?id=5
, which runsSELECT name, description FROM items WHERE id = 5;
. If the page for ID 5 loads, the attacker knows ID 5 exists. - Test for vulnerability:
https://example.com/item?id=5 AND 1=1
(Should load the same asid=5
if vulnerable and1=1
is true).https://example.com/item?id=5 AND 1=2
(Should result in an error or blank page if vulnerable and1=2
is false).
- Extracting information: Once vulnerability is confirmed, the attacker can extract data character by character or even bit by bit. For example, to find the first character of the database name:
https://example.com/item?id=5 AND SUBSTR(database(), 1, 1) = 'a'
(If the page loads, the first character is 'a')https://example.com/item?id=5 AND SUBSTR(database(), 1, 1) = 'b'
(If the page loads, the first character is 'b')- ... and so on, iterating through characters until the page indicates 'true'.
- This process is slow and requires many requests, but can be automated using tools.
- Example: Imagine a URL like
Time-Based Blind: This is used when the application's response is the same regardless of the injected boolean condition's result (e.g., always returns a generic error). Attackers inject SQL statements that cause a delay if a condition is true.
- Example: To check if the first letter of the database name is 'a':
SELECT ... WHERE id = 5 AND IF(SUBSTR(database(), 1, 1) = 'a', SLEEP(5), 0); --
- If the condition
SUBSTR(database(), 1, 1) = 'a'
is true, theSLEEP(5)
function is executed, causing the database query (and thus the page load) to take 5 seconds longer. - If the condition is false,
SLEEP(5)
is skipped, and the page loads quickly.
- If the condition
- By measuring the response time, the attacker can infer the truth value of the condition. This is even slower than boolean-based methods without automation, but effective when boolean differences are not observable.
- Example: To check if the first letter of the database name is 'a':
Blind SQL injection techniques, while seemingly tedious, are incredibly powerful when combined with automation tools like sqlmap, which can perform the hundreds or thousands of requests necessary to extract significant amounts of data once a blind vulnerability is identified.
4.3. Second-Order SQL Injection
This technique is more subtle and harder to detect with automated scanners alone. It occurs when malicious input isn't executed immediately, but is stored in the database, and then retrieved and used unsafely in a different part of the application later.
Definition: Second-Order SQL Injection occurs when an application stores unsanitized user input containing malicious SQL fragments in the database. The injection is then triggered later when another part of the application retrieves and uses this stored malicious data to build and execute a new, vulnerable SQL query.
Think of it like a time bomb. The attacker's input (' OR '1'='1 --
) might be entered into a profile "bio" field. The code handling the saving of the bio field might be secure (e.g., using parameterized queries). However, a different part of the application, like an admin panel that displays user bios by concatenating them into a query string for a reporting feature (SELECT * FROM users WHERE bio LIKE '%" + stored_bio + "%';
), might not use parameterized queries. When the admin views the report, the stored malicious bio gets injected into the vulnerable reporting query and executed within the admin's context, potentially granting the attacker access or executing commands with higher privileges.
Successfully exploiting second-order injection requires a deeper understanding of the application's data flow and how stored data is used in subsequent operations. This makes manual testing and source code analysis crucial for finding these vulnerabilities.
5. Real-World Consequences: When "Forbidden Code" Hits Hard
SQL injection isn't just theoretical; it has been the root cause of some of the most significant data breaches in history. These examples highlight the devastating impact this vulnerability can have:
- Guess.com (2002): An early high-profile case where an attacker stole over 200,000 customer records, including credit card data, via SQL injection.
- Rhode Island Government (2006): Credit card data stolen from users interacting with state agencies online.
- US Military Websites (2007, 2009): Websites belonging to the McAlester Army Ammunition Plant and the US Army Corps of Engineers exploited by Turkish hackers.
- Albert Gonzalez (Starting ~2005): A series of breaches affecting major companies like Heartland Payment Systems, 7-Eleven, and Hannaford Brothers, resulting in the theft of over 130 million credit card numbers using SQL injection. This was dubbed "the biggest case of identity theft in American history" at the time.
- The Pirate Bay (2010): Sensitive user information, including IP addresses and password hashes, obtained.
- TalkTalk (2015): A significant breach affecting a major British telecommunications company, compromising personal data of 400,000 customers, reportedly through an easily exploitable SQL injection vulnerability in a legacy system. This event drew criticism as such vulnerabilities were well-known and preventable.
- Gab (2021): A large data exfiltration from the social media platform attributed to an SQL injection vulnerability.
- MOVEit (2023): A widespread supply chain attack leveraging a critical SQL injection vulnerability in the MOVEit file transfer service, affecting numerous global organizations and leading to massive data theft.
- TSA FlyCASS (2024): An SQL injection found in a system used by the Transportation Security Administration, potentially allowing unauthorized access and modification of crew records (though mitigation layers reportedly reduced the immediate risk).
These examples underscore that SQL injection is not an abstract concept but a clear and present danger that continues to be exploited against organizations of all sizes, often with severe financial, legal, and reputational consequences.
6. Defense: Closing the Backdoor
Understanding how SQL injection works is the first step to defending against it. As attackers constantly probe for weaknesses, developers must build applications with strong security measures from the ground up. OWASP and security professionals advocate several core strategies to mitigate SQL injection risks.
6.1. Core Mitigation Strategies
These are the most effective and recommended defenses:
Parameterized Statements (Prepared Statements / Bind Variables):
Definition: Parameterized Statements separate SQL query code from user-supplied data. The database driver or ORM sends the query structure to the database first, which "prepares" it. Then, the data values are sent separately and bound to placeholders within the prepared query. The database understands that the bound values are purely data and should never be interpreted as executable SQL code.
- How it works: Instead of building a single string like
"SELECT * FROM users WHERE username = '" + user_input + "'"
, you define the query structure with placeholders (often?
or named parameters like:username
):String sql = "SELECT * FROM users WHERE username = ?"; PreparedStatement statement = connection.prepareStatement(sql); statement.setString(1, userInput); // Bind userInput as a string value ResultSet results = statement.executeQuery();
- If the
userInput
is' OR '1'='1'; --
, the database receives the query templateSELECT * FROM users WHERE username = ?
and the value' OR '1'='1'; --
for the placeholder. It will search for a username that literally matches the entire string' OR '1'='1'; --'
, which is highly unlikely to exist. The injected characters are treated as part of the data, not as SQL commands. - Why it's effective: It completely prevents the attacker's input from changing the query's structure or logic because the data is never concatenated directly into the command string. This is the primary and most recommended defense against SQL injection.
- How it works: Instead of building a single string like
Allow-List Input Validation:
Definition: Allow-list Input Validation (also known as positive validation) is a security practice where an application explicitly defines and accepts only known valid inputs, rejecting everything else. This contrasts with deny-list (or blacklist) validation, which tries to block known malicious patterns but is easily bypassed.
- How it works: Before using user input in a query, check if it strictly conforms to the expected format, type, length, and character set. For example:
- If an input should be a user ID, validate that it is a positive integer.
- If an input should be an email address, validate it against a strict email format regex.
- If an input should be a date, parse it strictly according to the expected date format.
- Why it's a supplementary defense: While it doesn't directly prevent the query construction issue, it can filter out malformed or unexpected input that might contain injection attempts before it even reaches the vulnerable query building code. It's a strong layer of defense but should not be relied upon as the only defense against SQLI, as complex or novel injection strings might still conform to basic validation rules but exploit weaknesses in query construction.
- How it works: Before using user input in a query, check if it strictly conforms to the expected format, type, length, and character set. For example:
Least Privilege:
Definition: The principle of Least Privilege dictates that any user, program, or process should have only the minimum necessary permissions to perform its intended function.
- How it works in databases: The database user account that your application uses to connect to the database should have only the specific permissions required by the application.
- If the application only needs to read data, the user account should have only
SELECT
permissions. - If it needs to add users, it should have
INSERT
permissions on theusers
table, but notDELETE
orDROP TABLE
. - Application accounts should almost never have administrative privileges (
CREATE TABLE
,ALTER TABLE
,DROP TABLE
, access to system tables, etc.).
- If the application only needs to read data, the user account should have only
- Why it's effective: Even if an attacker successfully injects malicious code (e.g.,
'; DROP TABLE users; --
), the database will refuse to execute theDROP TABLE
command if the application's database user account lacks that permission. This limits the potential damage an attacker can inflict, preventing total database compromise or destruction.
- How it works in databases: The database user account that your application uses to connect to the database should have only the specific permissions required by the application.
6.2. Supplementary Mitigation: Object-Relational Mappers (ORMs)
Definition: An Object-Relational Mapper (ORM) is a programming tool or framework that provides an object-oriented interface to a relational database. It allows developers to interact with database tables and rows using objects and methods in their programming language, abstracting away much of the direct SQL interaction.
- How they help: Most modern ORMs (like Hibernate for Java, SQLAlchemy for Python, Entity Framework for .NET, Eloquent for PHP Laravel) use parameterized queries under the hood when you use their standard methods to query, insert, or update data based on objects. This means that data passed through standard ORM methods is usually handled securely, preventing injection.
- Caveats: ORMs are not a guarantee against SQL injection. Developers can often bypass the ORM's safe methods by executing raw SQL queries directly through the ORM interface. If these raw queries are constructed using string concatenation with unsanitized user input, the ORM provides no protection, and the application remains vulnerable. Relying solely on using an ORM without understanding how it handles queries and when it doesn't provide protection can lead to a false sense of security.
6.3. Deprecated/Secondary Approaches: String Escaping
Historically, one method to prevent SQL injection was String Escaping.
Definition: String Escaping is the process of modifying special characters within a string so that they are treated as literal characters rather than having their special meaning in the context of the query.
- How it worked: Developers would iterate through user input strings and add an "escape" character (like a backslash
\
) before any character that had special meaning in SQL string literals (primarily the single quote'
, but sometimes backslash\
, double quote"
, or others depending on the specific database and its configuration). For example, if the user enteredO'Malley
, it would be escaped toO\'Malley
. When inserted into the query string"SELECT ... WHERE name = '" + escaped_input + "'
, it would become"SELECT ... WHERE name = 'O\'Malley'"
. The database would see the\'
as an escaped literal quote, not the end of the string, correctly interpreting the input as the literal name. - Why it's deprecated: OWASP and security experts strongly discourage string escaping as a primary defense because it is "frail" and error-prone:
- Incomplete: It's easy to miss escaping one or more special characters, immediately re-introducing the vulnerability.
- Database-Specific: Special characters and escape methods vary between different database systems (MySQL, PostgreSQL, SQL Server, Oracle), making the code less portable and requiring careful adherence to specific database manuals.
- Context-Dependent: Escaping only works for data used within string literals. It offers no protection if user input is used in other contexts within the query, such as table names, column names, or numeric values in clauses like
LIMIT
orORDER BY
. For example, injecting into a column name context:SELECT data FROM user_table ORDER BY user_input;
whereuser_input
is'age'
. An attacker could enterage; --
or evenage DESC
. Escaping quotes wouldn't help here.
- Conclusion: While some functions like
mysqli_real_escape_string()
exist, they are part of older or less secure paradigms. Parameterized statements handle escaping automatically and correctly for the specific data type and database, in addition to separating data from code, making them vastly superior and the recommended approach.
7. In Popular Culture
SQL injection's blend of technical exploit and often simple vector has led to it appearing in various forms of media, making it one of the more widely recognized cybersecurity concepts:
- "Bobby Tables" (xkcd comic): A famous 2007 webcomic by Randall Munroe featured a mother getting a call from her child's school because her son, named
Robert'); DROP TABLE Students;--
, had disrupted their database. This comic popularized SQL injection and led to the informal nickname "Bobby Tables" for injection attacks. - J.K. Rowling's "The Casual Vacancy" (2012): The novel included a subplot where characters perform unauthorized logins to a website using SQL injection.
- Dariusz Jakubowski x'; DROP TABLE users; SELECT '1 (2014): A Polish individual legally changed his business name to this string, humorously attempting to disrupt bots designed to harvest business names from websites, echoing the Bobby Tables concept in the real world.
8. Conclusion
SQL Injection remains a potent weapon in the attacker's arsenal because it targets the fundamental interaction point between applications and their data. It thrives on the simple mistake of treating untrusted input as if it were internal code. While complex attack techniques like Blind and Second-Order injection demonstrate the lengths attackers will go, the most successful exploits often target basic vulnerabilities arising from inadequate input handling and query construction.
Understanding SQL injection is essential for anyone working with data-driven applications. For those exploring the "forbidden code," it reveals how a seemingly small programming oversight can open a back door to an entire database. For those building applications, it highlights the critical need for secure coding practices, particularly the use of parameterized statements, strict input validation, and the principle of least privilege. By applying these defenses rigorously, you can protect your data and your users from one of the internet's most enduring threats.